Building a Gift Card Feature for an iOS App
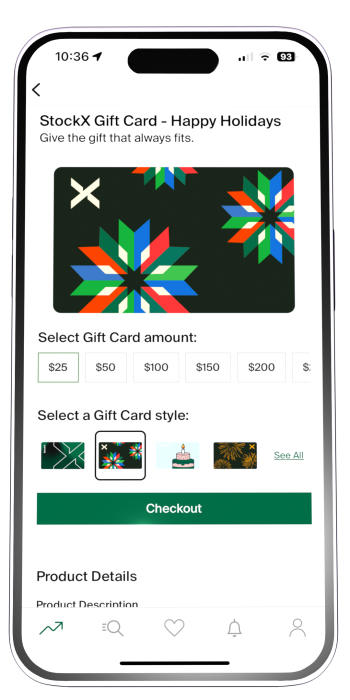
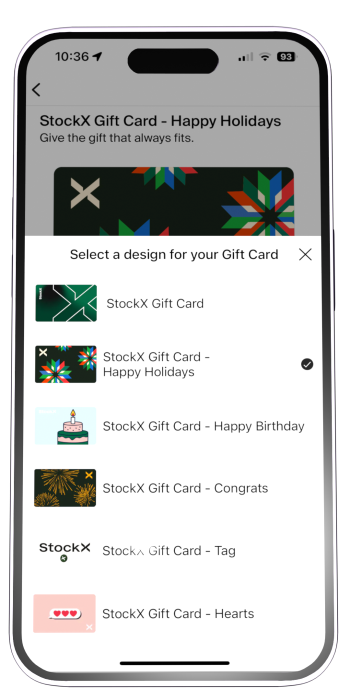
Disclaimer: The following explanation describes a sample implementation of a gift card feature for an iOS app similar to StockX. It is not the actual code used by StockX but rather a conceptual example.
In this post, we’ll go over how to build a gift card feature for an iOS app like StockX. The feature will allow users to view and interact with a set of gift cards, offering an intuitive browsing experience. Initially, four gift cards will be displayed on the main screen, and upon tapping a “View All” button, users will be navigated to a separate screen displaying a full list of 20 gift cards, with a pagination system to navigate through them.
Step 1: Setting Up the Project
Start by creating a new iOS project and setting up the necessary view controllers:
- MainViewController – The first screen that displays four gift cards.
- GiftCardsListViewController – The second screen that shows all 20 gift cards with pagination.
The key data structure for this feature will be a simple gift card model, which could later be populated from an API or other data source.
Step 2: Define the Gift Card Model
Each gift card can have basic properties such as an ID, title, and value. These properties can be used to represent the information associated with each card. While the data for the gift cards in this example will be hardcoded, it can easily be adapted to fetch data dynamically from a backend or API in a real-world scenario.
Step 3: Designing the Main View
The MainViewController is responsible for displaying the first four gift cards in a collection view. The collection view allows users to scroll through the gift cards, and a “View All” button provides a way to navigate to a new screen.
When users tap the “View All” button, they will be presented with the GiftCardsListViewController, which shows a list of all 20 available gift cards.
Step 4: Implementing the Full List View
The GiftCardsListViewController will display the full set of gift cards, but instead of showing them all at once, the feature uses pagination to make the list easier to navigate. A UIPageViewController is the ideal choice here. This component allows users to scroll horizontally between pages, each page containing a single gift card. As users swipe, they can view all 20 gift cards without being overwhelmed by a long, static list.
The GiftCardsListViewController will act as the data source and delegate for the UIPageViewController, managing the transition between pages and displaying the appropriate gift card for each page.
Step 5: Gift Card Page View Controller
Each page in the UIPageViewController will be represented by a separate view controller that displays a single gift card’s information. The page view controller will render the title and value of the gift card, providing a detailed view as users swipe through the cards.
This view controller will also include navigation logic to allow the user to move between pages seamlessly, ensuring a smooth and intuitive experience.
Step 6: Designing the User Interface
On the main screen, the MainViewController will contain a UICollectionView that shows the first four gift cards. The user can scroll through the cards horizontally or vertically, depending on how the collection view is configured. The “View All” button will be prominently displayed at the bottom of the collection view.
On the second screen, the GiftCardsListViewController will utilize the UIPageViewController to display each gift card in a paginated view. The user will be able to swipe left or right to navigate between cards.
Step 7: Testing the Feature
Once the user interface and functionality have been implemented, it’s time to test the feature. First, ensure that the main view displays four gift cards as expected. After tapping “View All,” the GiftCardsListViewController should be presented, showing all 20 gift cards in a paginated view. Users should be able to swipe through the cards smoothly, and the data for each card should be displayed correctly.
Conclusion
This implementation offers a user-friendly way for users to interact with gift cards on the StockX iOS app. By combining a collection view for the initial display and a paginated page view controller for the full list, users can easily navigate through the cards without feeling overwhelmed. The design is scalable, and this approach can be adapted to other features or collections of items in the app.
This gift card feature provides a clean and intuitive user experience, with room for expansion as the product evolves. Whether the data is fetched from an API or a local database, the structure we’ve discussed here allows for easy integration of additional functionality as needed.